Android CheckBox Example : Getting started with Android
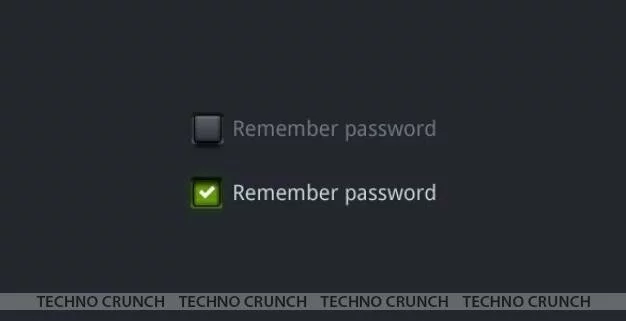
Android checkbox is used to select more than one option at a time.
Ex: – In an admission form we may need to select both diploma & BE in Graduate option. For this we can use checkbox which will enable us to select multiple options.
CheckBox Example: –
Your XML code should look like
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
|
xml version="1.0" encoding="utf-8"?>
LinearLayout android:id="@+id/LinearLayout01" android:layout_width="fill_parent"
android:layout_height="fill_parent" xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical">
CheckBox android:id="@+id/check1"
android:layout_width="wrap_content"
android:layout_height="wrap_content" android:text="Android" />
CheckBox android:id="@+id/check2"
android:layout_width="wrap_content"
android:layout_height="wrap_content" android:text="iPhone" />
Button android:id="@+id/button1"
android:layout_width="wrap_content"
android:layout_height="wrap_content" android:text="Confirm Selection"/>
TextView android:id="@+id/TextView01" android:layout_width="wrap_content"
android:layout_height="wrap_content" android:text="Selected is" />
EditText android:id="@+id/text1"
android:layout_width="wrap_content"
android:layout_height="wrap_content" android:text="EditText"
android:textSize="18sp"/>
/LinearLayout>
|
Your Java code should look like
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
|
public class CheckBoxExample extends Activity implements Button.OnClickListener
{
Button b1;
CheckBox c1, c2;
EditText et1;
@Override
public void onCreate(Bundle icicle)
{
super.onCreate(icicle);
setContentView(R.layout.main);
et1 = (EditText) this.findViewById(R.id.text1);
c1 = (CheckBox) findViewById(R.id.check1);
c2 = (CheckBox) findViewById(R.id.check2);
b1 = (Button) findViewById(R.id.button1);
b1.setOnClickListener(this);
}
@Override
public void onClick(View arg0) {
// TODO Auto-generated method stub
et1.setText("");
if (c1.isChecked())
et1.setText("Android ");
if (c2.isChecked())
et1.setText(et1.getText()+"iPhone ");
}
}
|
The output will look like

